IoT — Reading and sending sensor data
Author
KalleDate Published
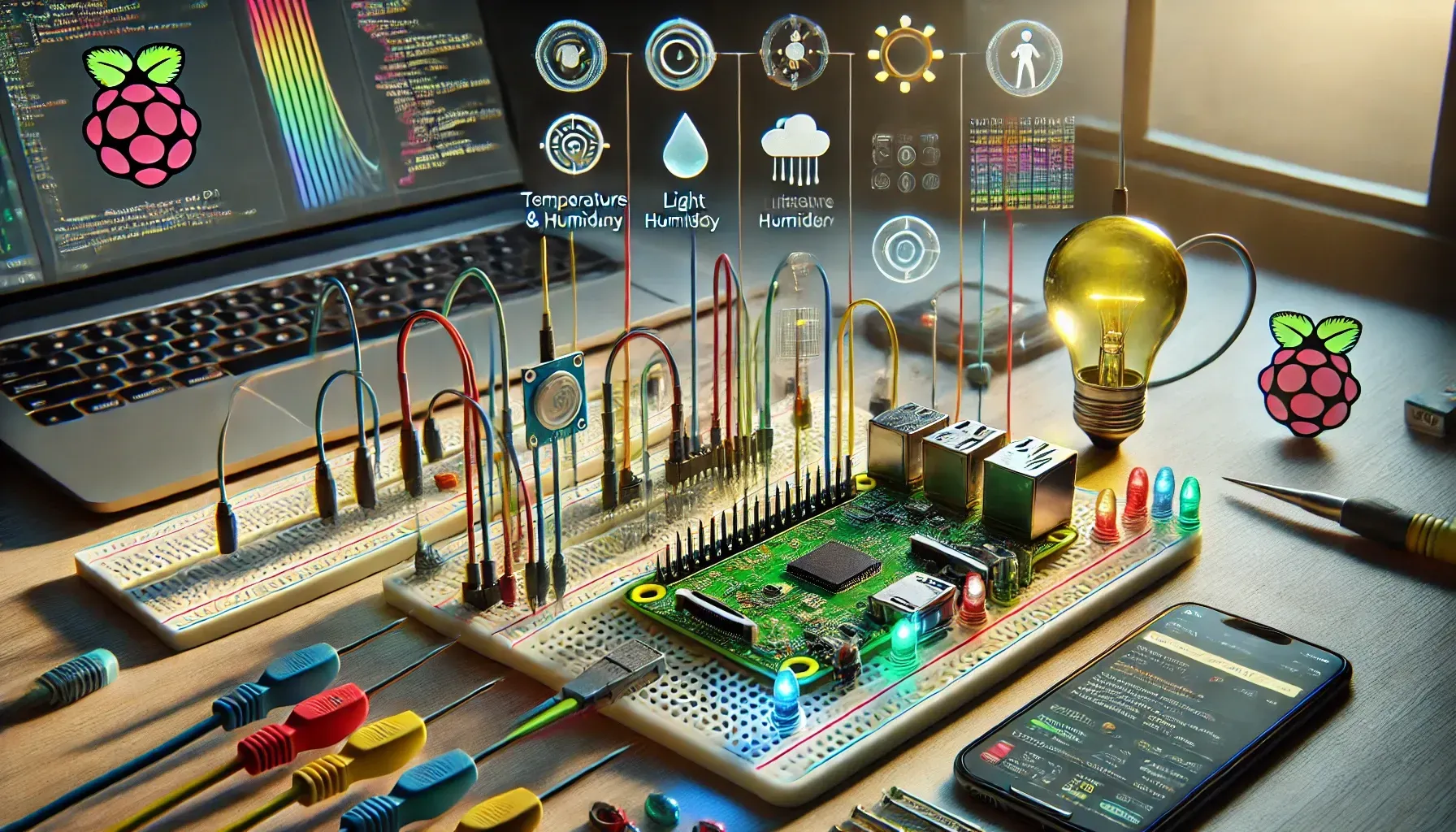
One of the most common use cases of IoT is reading and collecting sensor data. Here I will show an example using the DHT11 sensor which reads the temperature and humidity values in its environment.
A simple setup would look like this, the sensor is connected with its data pin to the GPIO pin 4 and an error led is connected to the GPIO pin 23, it shall blink when there is an error.
To address the sensor from node there is another dependency which has to be installed. The node-dht-sensor
package uses the BCM2835 library. To install this library connect to your Raspberry Pi via ssh and start by downloading the current library version (1.57) after downloading unpack and install it:
With the BCM2835 library installed the project setup can continue. As you already did for the blinky app create a new folder ~/sensors01
in your home folder on the Raspberry Pi. This folder will be used as target to transfer the files over scp
.
On your normal computer start a new project and add a package.json
:
The listed dependencies are :
- axios - a package for easier http requests
- node-dht-sensor - used to read the DHT sensor
- onoff - for easy access to the gpio pins
- sleep-promise - awaitable sleep function
- yargs - simplifies access to arguments used to start the program
As a start for your sensor reading program you can use this code:
Now it's time to bring some internet to the things, the program above needs a server to send the collected data to. For an easy start the server should be as simple as possible. I recommend using a library made for micro-services called micro.js, it lets you build single purpose servers easily.
To begin working on the server create another project and start with this package.json:
The listed dependencies are :
As a starting point for the server you can use the following script:
Tasks
- prepare the Raspberry Pi with the installation of the BCM2835 library
- connect a DHT11 sensor with the Raspberry Pi
- connect a LED with the Raspberry Pi as an error signal
- extend the program running on the RPi to read temperature and humidity values from the DHT11 sensor
- create a server to collect data posted to it
IoT — Communication protocols
Explore the various communication protocols used in IoT, focusing on MQTT for efficient machine-to-machine communication. This guide explains MQTT's lightweight binary protocol, its publish/subscribe model, and how to implement it using a message broker and Node.js.
Mailing List
If you want to receive updates on new posts, leave your email below.