Project WebApp — Functional programming with JavaScript
Author
KalleDate Published
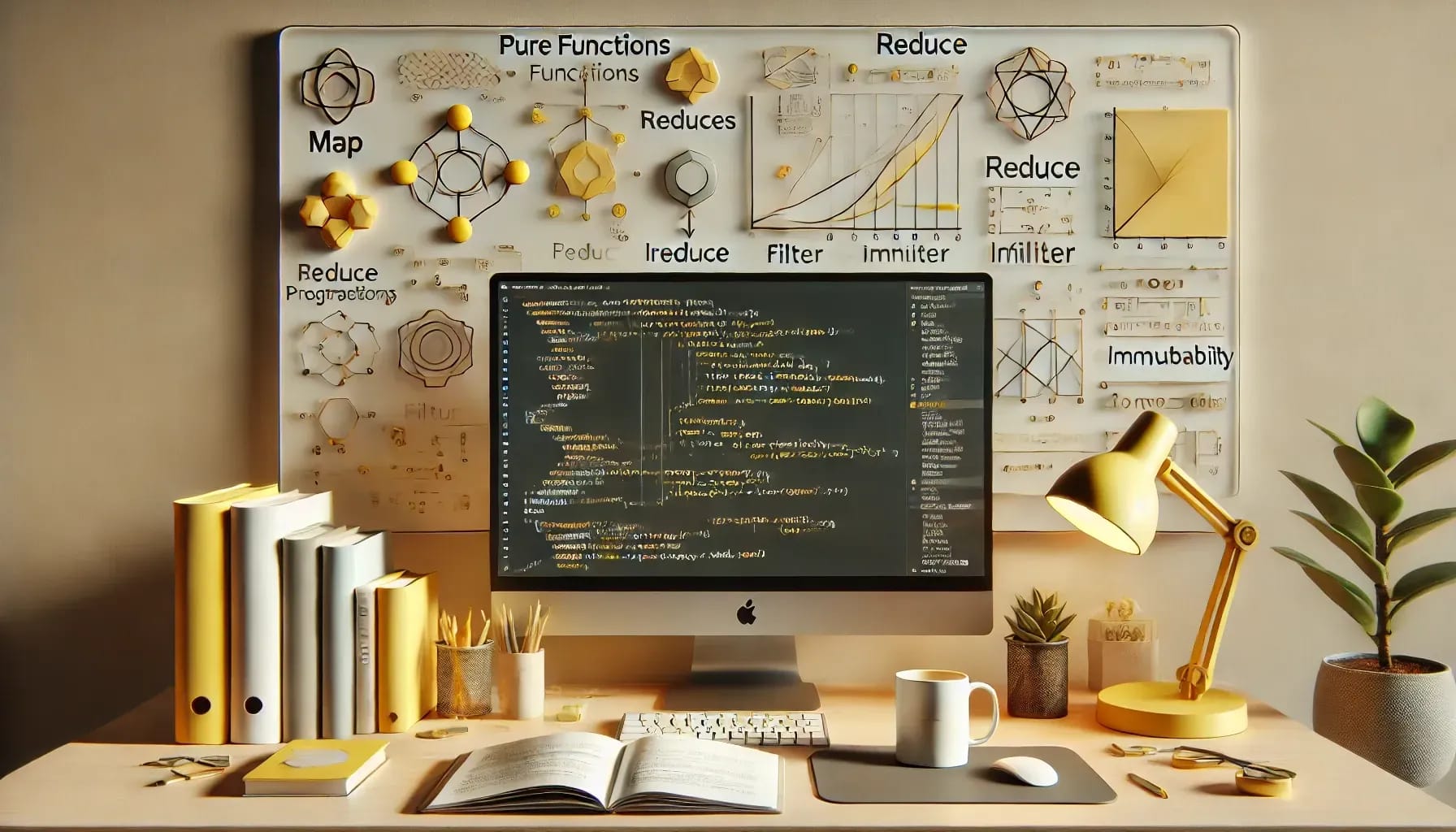
As the name "functional programming" suggests, this lesson has a heavy focus on functions. In JavaScript, you can describe a function in several ways:
- with the
function
keyword:
- as a method (same behavior as the function keyword):
- store it as an anonymous function in a variable
- and the best part: define it as an arrow function
Arrow functions have some benefits over the classical function/method declaration.
- the
this
context gets set at the time of definition - it's a bit shorter to write (with only one parameter you can even omit the parentheses)
A short excursion on why this
is weird in JS:
In the programming model of JavaScript it is totally fine to redefine the owner of a function when you call it, so whenever you use this
, you have to make sure that the context is set correctly when you call the function.
A short example:
To solve this, like always, you have several possible ways to go.
- Avoid this-context by using direct access to the owning object (BAD):
- Use a class and arrow-function for a fixed this-context:
- Go functional and use an independent greeting function:
The lesson-learned should be, only use this
if you really have to, when possible use pure functions.
Now back to functional programming.
One fundamental building block is the usage of "pure" functions, this definition is close to the mathematical definition of functions f(x) -> y
. A pure function returns a value purely defined by the parameters passed into it, and there should be no dependencies to variables defined outside of the function's scope. The returned value is also always the same if the passed parameters are the same (no randomness or hold state in the function). Besides calculating the resulting value the function has no other effects. So never ever mutate the incoming values.
pure or impure?
purepure or impure?
impurepure or impure?
impurepure or impure?
purepure or impure?
impurepure or impure?
pure
Another very important paradigm in functional programming is "declarative programming". Instead of describing the control flow of the program you should care about the logic and behavior. For example use recursion instead of loops.
Task
- write a pure and declarative function calculating of the fibonacci sequence as a start use this imperative and impure implementation:
Here is a codesandbox with tests to work on this in the browser: Edit Fibonacci
Passing functions around
The parameters passed into a function can be data, like numbers, booleans, strings, objects... and also functions. So you can call a function with a function. Even the returned value can be a function.
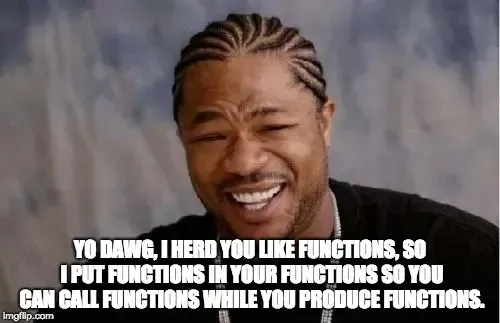
Functions taking other functions as arguments or returning functions are called "higher-order-functions"
Getting back to the greeting function, this pattern allows you to define a function to return a function to generate a greeting message for a specific name.
or in a shorter way:
The most commonly used higher-order-functions in JS are array-functions like forEach
, filter
, map
and reduce
. Those functions take a function as input and call this function with every element of the array.
Examples:
Edit Star Wars API - data transform exercises
Tasks:
- get familiar with the array functions
- get all starships with a hyperdrive rating over 2 which appear in the movie "A new Hope"
- are there any transports capable of carrying more than 8 passengers which are cheaper than 400000 credits
- how fast is the TIE bomber?
- how many characters are listed for the movie "The Phantom Menace"?
- list all the characters from the movie "Revenge of the Sith" matched to their species
- what is the longest opening crawl of all movies?
Project WebApp — From code to UI - Introduction to React.JS
Get started with React by learning to create UI components using JSX. This introduction covers the basics of setting up a React environment, creating simple components, and understanding JSX syntax. Learn how to build interactive web applications efficiently with practical examples.
Mailing List
If you want to receive updates on new posts, leave your email below.