Project WebApp — Declarative animations with react-pose
Author
KalleDate Published
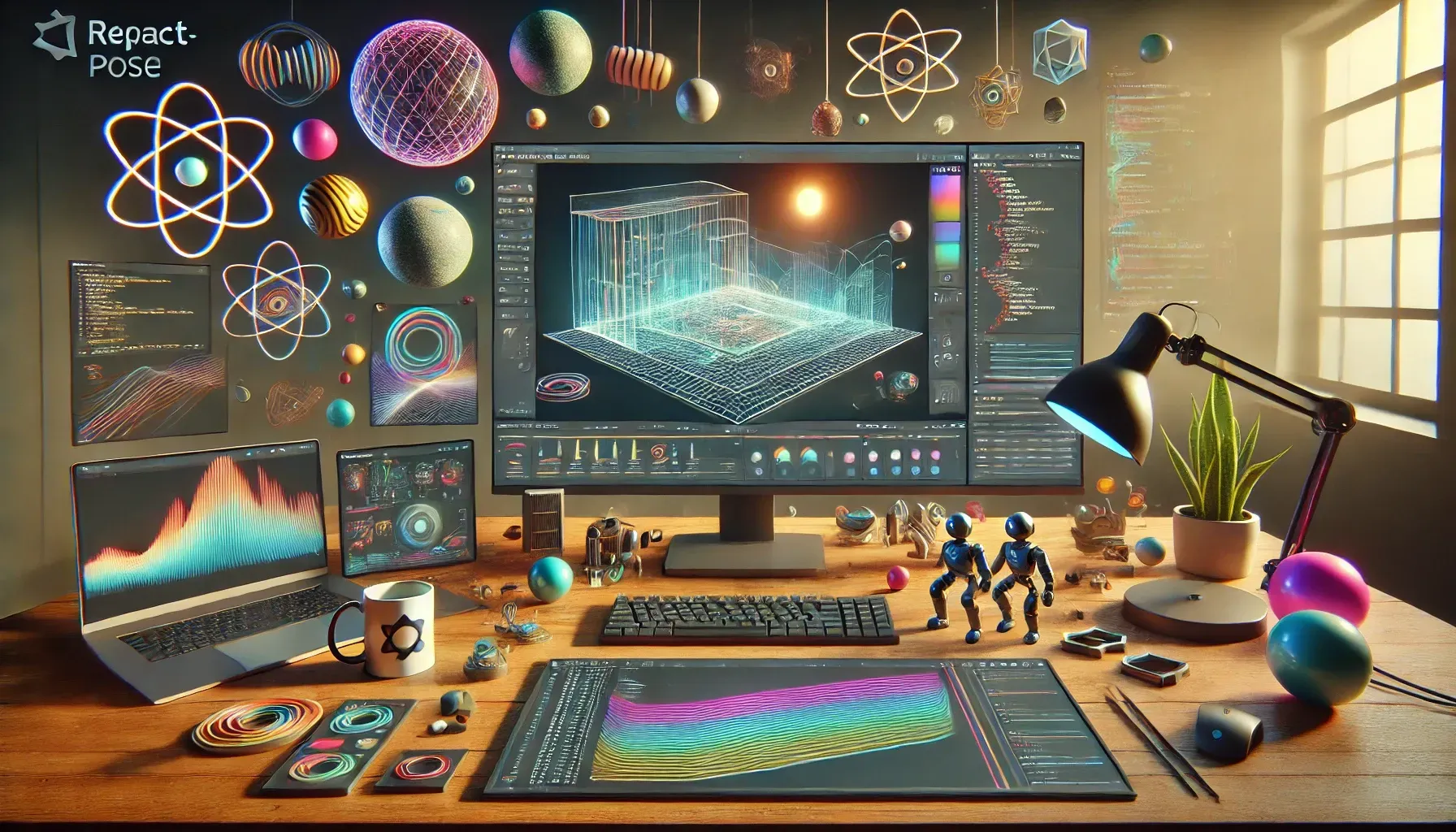
In general animating the view is hard, because suddenly the state is dependent on time. Views can exist longer than they existed before because of exit animations, or some layouts must wait for other elements to take their place. And animations are expensive, so don't do too much and whenever possible you should use hardware accelerated key-frame animations or transitions. But sometimes the animations get a little more complex and are difficult to handle with pure CSS and style rules. Then it's time to take some animation library to help.
One of the large goals of react is to describe as much of the app in a declarative way, for styling the styled-components workflow is working really good and react-pose has a similar API to animate components. Instead of styles you define different "poses" the component can have and define how the transitions of values between the poses should work.
For example a sidebar component which can be hidden on the left side of the screen would look like this:
If you want to have enter and exit animations you need a Wrapper Component managing newly created and disappearing components. With react-pose you can use the PoseGroup
to manage posed components. Every direct child of a PoseGroup will get the poses enter
and exit
and needs a unique key
prop to enabled tracking of the elements.
As an exercise try to rebuild this animation from dribble, a working result could look like this:
video:
You can use this repository as a basis.
Tasks
- identify all moving elements in the dribble design
- define poses for all UI states
- define transitions for all pose changes
Project WebApp — React on the server and the JAMstack
Learn how to render React components on the server using react-dom/server and frameworks like Next.js. This guide also explores the JAMstack architecture, highlighting tools such as Gatsby for creating static sites with dynamic capabilities.
Project WebApp — Get firebase to sync your data
Learn how to sync data in your web app using Firebase's realtime database. This guide covers setting up Firebase, configuring anonymous authentication, and using Firebase to manage and sync data efficiently across clients.
Project WebApp — Taking control over state-changes
Explore how to manage state changes in JavaScript using RxJS. This guide introduces observables, subjects, and operators like map and catchError, showing how to handle data streams efficiently and integrate them with React components.
Project WebApp — State management in a react app
Learn to manage state in React using built-in state APIs, focusing on immutability and single sources of truth. This guide covers setState basics, best practices, and introduces Redux for complex state management with practical examples
Project WebApp — How to style react
Learn how to style React components using various approaches, including inline styles, styled-components, glamorous, and emotion. This guide explains each method's benefits and provides practical examples to help you implement component-based styling efficiently in your React applications.
Project WebApp — From code to UI - Introduction to React.JS
Get started with React by learning to create UI components using JSX. This introduction covers the basics of setting up a React environment, creating simple components, and understanding JSX syntax. Learn how to build interactive web applications efficiently with practical examples.
Project WebApp — Functional programming with JavaScript
Learn the basics of functional programming in JavaScript, including pure functions, higher-order functions, and declarative programming. This guide offers practical examples and exercises to help you create efficient, maintainable code.
Project WebApp — Basics and Tools
First steps in web development: learn the basics of HTML, CSS, and JavaScript, and essential tools like Babel, Webpack, and Node.js. Set up a development environment with Visual Studio Code, ESLint, Prettier, and Git. These fundamentals ensure efficient web application development.
Mailing List
If you want to receive updates on new posts, leave your email below.